In the days of Z80 and 6502 programming in low memory systems self modifying code was quite common. Not only was it extremely efficient space and speed wise, it often was the only real way to get things done in systems with nothing but a few kb of memory. Explaining the concept of self modifying code is quite simple, and basically everyone can grasp it in a minute.
Enter the dreaded word recursion.
When recursion is mentioned it is all too often matched with a blank gaze and sweaty palms. Getting it explained is possibly even harder from a non hardcore programmer. Yet is a concept that matches self modifying code in its simplicity.
Self calling code.
Once explained along the lines of a block of code calling itself to get something done, the whole thing all of a sudden changes from black magic to an easy to grasp concept. Don’t ask me _why_, i just observed the effects a few too many times 🙂
whereas there are good alternatives for recursion (sorry-) self calling code – like using a stack object (see this document ) recursion often is the most efficient way to get things done.
So without further ado, i give you one more self calling code sample thats is much more straightforward compared to this document: Populating a DX Treelist control from code or data .
The code below populates a asp.net tree list with category data. Nested category data. Consider a structure like this:
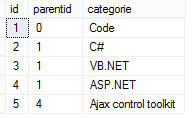
The idea is simple, A parent id of 0 means it is a root category at the top. Any other value nests the category below the one we point to.
The implementation is equally simple. All we have to is is scan the top level and then have a function scan anything that points to it. And that is where the self calling element comes in. Any sub item that is found, is passed right back in to be scanned itself. And any item found on that one is passed back in, and on, and on. The scanner code is recursively calling itself, and thus walking the tree for us.
Thats all there really is to it 🙂
/// <summary> /// Bind the rootlevel node items /// </summary> private void BindCatList() { var topCat = from c in _dc.Categories where c.Parentid == 0 orderby c.Categorie select c; foreach (var catItem in topCat) { int catId = catItem.Id; var rootNode = new TreeNode(catItem.Categorie, catItem.Id.ToString()); TreeViewCategories.Nodes.Add(rootNode); AddChildNodes(rootNode, catId); } } /// <summary> /// Recursively bind descending nodes /// </summary> /// <param name="node"></param> /// <param name="nodeId"></param> private void AddChildNodes(TreeNode node, int nodeId) { var catChildren = from c in _dc.Categories where c.Parentid == nodeId select c; foreach (Categories catItem in catChildren) { var newNode = new TreeNode(catItem.Categorie, catItem.Id.ToString()); node.ChildNodes.Add(newNode); AddChildNodes(newNode, catItem.Id); } }