The Ajax Control Toolkit offers a large amount op ASP.NET Ajax control, and can be found on codeplex. WatiN is a popular test framework for UI testing ASP.NET web applications. Both can be obtained from the linked site, or can easily be added in Visual Studio with nuget.
The objective of this writeup is to use WatiN to start an IE session, fire up a local website with the tab control on it, and proceed to iterate through the tab pages. This will result in a practical example of the usage of both. The reference solution containing the website and the WatiN project can be downloaded right here: AjaxTabs.zip After extracting the files and opening the solution “enable nuget package restore” and perform a build to retrieve and install the relevant dependency packages.
First off, we need to setup a working tab control. The markup as shown below consists of the tab container itself, and three tab panels. Each have an added ContentTemplate section which will contain your controls. A nice extra for the tab control from the Ajax Control Toolkit is that each control still can be directly referenced from code, and you do not need to use FindControl(…) or the likes.
Tab control demo markup
<%@ Page Language="C#" AutoEventWireup="true" CodeBehind="Default.aspx.cs" Inherits="AjaxTabs.Default" %> <!DOCTYPE html> <html xmlns="http://www.w3.org/1999/xhtml"> <head runat="server"> <title></title> </head> <body> <form id="form1" runat="server"> <div> <!-- script manager --> <ajaxToolkit:ToolkitScriptManager ID="ToolkitScriptManager1" runat="server"> </ajaxToolkit:ToolkitScriptManager> <!-- tab control --> <ajaxToolkit:TabContainer ID="TabContainer1" runat="server" ActiveTabIndex="0"> <ajaxToolkit:TabPanel runat="server" HeaderText="TabPanel1" ID="TabPanel1"> <ContentTemplate> Textbox: <asp:TextBox runat="server" ID="TextBoxDemo"></asp:TextBox> <asp:Button runat="server" ID="ButtonTest" Text="ClickMe" OnClick="ButtonTest_Click"/> </ContentTemplate> </ajaxToolkit:TabPanel> <ajaxToolkit:TabPanel runat="server" HeaderText="TabPanel2" ID="TabPanel2"> <ContentTemplate> <h2>Panel 2 content</h2> </ContentTemplate> </ajaxToolkit:TabPanel> <ajaxToolkit:TabPanel ID="TabPanel3" runat="server" HeaderText="TabPanel3"> <ContentTemplate> <h2>Panel 3 content</h2> </ContentTemplate> </ajaxToolkit:TabPanel> </ajaxToolkit:TabContainer> </div> </form> </body> </html>
When run, this will look like this:
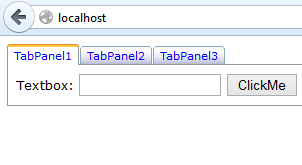
Using WatiN to test
Next we need a simple solution to actualy perform the tests. WatiN works in my experience reliable with IE9, is unstable with IE10 and simply does not work with IE11, so my advice is to use a Windows 7/IE9 combination to perform the tests. A virtual machine will do just fine. The test is embeeded in a simple console application which is marked with the STATHREAD attribute. You may initialy run into errors claiming Interop.SHDocVW or Microsoft.mshtml are not present if you from outside VS.This can simply be resolved by setting the Embed Interop Types setting to False and Copy Local to True. The code below is very linear. A web browser is started and attached to the page. In a loop the three tab pages are located withing the browser and clicked. Finaly, the browser is closed and the application ended.
// // Filename: Program.cs // Project: WatinTabs // Solution: AjaxTabs // // Created 2014-01-30 13:13 // Last modified: 2014-01-30 16:23 using System; using System.Threading; using WatiN.Core; namespace WatinTabs { internal class Program { [STAThread] private static void Main(string[] args) { var testBrowser = new IE("http://z400.corp.nhcoding.nl"); // point to your publish location for (int i = 0; i < 10; i++) { // // Find and click Tab 1 var tabLink = testBrowser.Link(Find.ById("__tab_TabContainer1_TabPanel1")); tabLink.Click(); Thread.Sleep(500); // // Find and click tab 2 tabLink = testBrowser.Link(Find.ById("__tab_TabContainer1_TabPanel2")); tabLink.Click(); Thread.Sleep(500); // // Find and click tab 3 tabLink = testBrowser.Link(Find.ById("__tab_TabContainer1_TabPanel3")); tabLink.Click(); Thread.Sleep(500); } Console.Write("end-run."); Console.ReadLine(); testBrowser.Close(); } } }
If you get serious with WatiN it would be wise to setup your test in such a way that each test checks only one page. That way you can even with a large application keep a good structure of folders representing your application structures that contain the tests. In a situation like this tab control, a page test for the page containing the control, and separate tests for each tab page could be an efficient setup.