Objective
Converting a nested data class to and from JSON, using the NewtonSoft JSON library.
Baseline
JSON is a text like format that can easily be created in a number of ways. However, you should try to restrain the reflex to simply use a stringbuilder for small operations, since these things tend to grow and you may likely end up with tens of lines outputting a slew of { and ” characters to make something resembling valid JSON.
In the .net world, the go to library is Newtonsoft.Json, so we will be using that. For other methods of creating Json take a look here for an idea of how easy the Newtonsoft solution actually is. The code sample below shows the framework of the test case, having only the data structure that will be used and nothing else. We will be using a customer object that can have multiple adresses nested. The nesting level has been kept low for demonstration purposes, there is no real limit to how complex you can make your object.
using System.Collections.Generic; namespace JSON_Sample { internal class Program { #region Procedures private static void Main(string[] args) { var custDemo = new Customer() { Name = "John Doe", Adresses = new List<Address>() { new Address() { Street = "Somestreet", House = "10", Postalcode = "1234" }, new Address() { Street = "OtherStreet", House = "20", Postalcode = "4567" } } }; } #endregion #region Nested type: Address public class Address { public string Street { get; set; } public string House { get; set; } public string Postalcode { get; set; } } #endregion #region Nested type: Customer public class Customer { public string Name { get; set; } public List<Address> Adresses { get; set; } } #endregion } }
Serializing and deserializing
Now instead of a while slew of code that you may expect, creating Json form the object takes a whole 1 line of code, and converting Json back into a new object takes another 1 line of code. That means that the real takeway here is that you should never bother with manual Json processing at all, represent your data with a simple class and be done with it.You will thank yourself when any kind of maintenance will need to done at all (compare adding a nested field somewhere in a manually created stringbuilder structure vs. adding a field to your data class)
// // Convert the customer to Json string json = JsonConvert.SerializeObject(custDemo); // // Create a new customer form the Json string var newCust = JsonConvert.DeserializeObject<Customer>(json);
The serialize command simply takes your object, figures out what the structure is, and creates Json from it. The contect of string string looks like this:
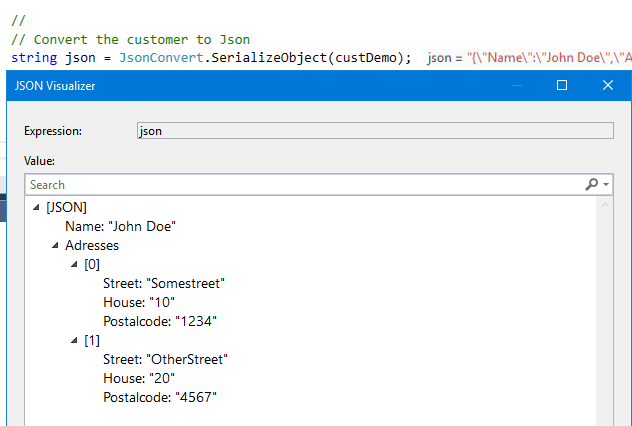
The magic in the deserialize command is that the data structure that we want to receive the data is passed in ( ), so the library knows exactly what to expect and create. The newly created object show up in the debugger like this:
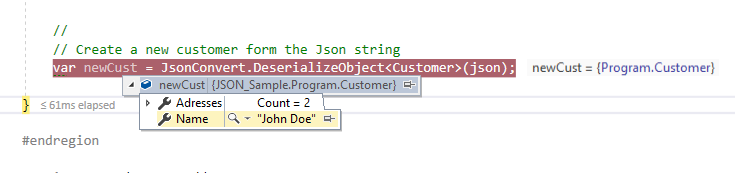
This is absolutely all you need to convert json to objects and back. The home of this great library can be found here
Full listing
After adding the Newtonsoft library from the nuget package manager, the full code looks like this
using System.Collections.Generic; using Newtonsoft.Json; namespace JSON_Sample { internal class Program { #region Procedures private static void Main(string[] args) { var custDemo = new Customer() { Name = "John Doe", Adresses = new List<Address>() { new Address() { Street = "Somestreet", House = "10", Postalcode = "1234" }, new Address() { Street = "OtherStreet", House = "20", Postalcode = "4567" } } }; // // Convert the customer to Json string json = JsonConvert.SerializeObject(custDemo); // // Create a new customer form the Json string var newCust = JsonConvert.DeserializeObject<Customer>(json); } #endregion #region Nested type: Address public class Address { public string Street { get; set; } public string House { get; set; } public string Postalcode { get; set; } } #endregion #region Nested type: Customer public class Customer { public string Name { get; set; } public List<Address> Adresses { get; set; } } #endregion } }