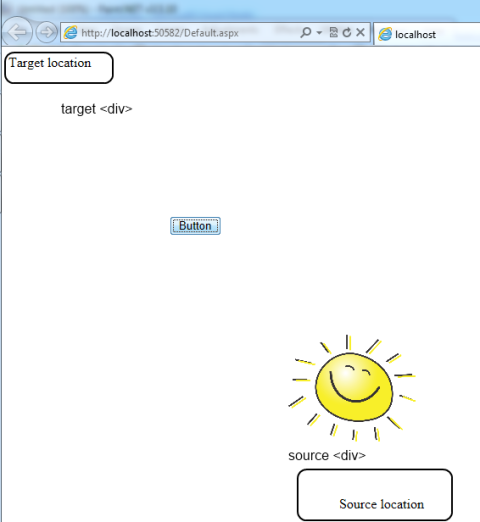
This sample animates an image from one position to another on the click of a button. JQuery is used to perform the animation. The idea is pretty simple. the animatesource div ands animatetarget div’s are placed on a page. The actual animation calculates the difference between the position of the tow div’s on the screen, and starts the JQuery animation from the target to the source.
<%@ Page Language="C#" AutoEventWireup="true" CodeBehind="Default.aspx.cs" Inherits="JSAnimation.Default" %> <!DOCTYPE html> <html xmlns="http://www.w3.org/1999/xhtml"> <head runat="server"> <title></title> <script type="text/javascript" src="jquery-1.9.1.js"></script> </head> <body> <form id="form1" runat="server"> <div> <div id="animatetarget" style="position: absolute; left: 10px; top: 10px;">Target location</div> <div id="animatebutton" style="position: absolute; left: 200px; top: 200px;"> <asp:Button ID="Button1" runat="server" Text="Button" OnClientClick="return false;" /> </div> <div id="animatesource" style="position: absolute; left: 400px; top: 400px;"> <img id="sun" src="Sun.png" width="150" alt="" style="position: relative;" /> Source location </div> </div> </form> <script type="text/javascript"> var buttonHandle = document.getElementById('<%=Button1.ClientID %>'); var sourcePos = $('#animatesource').position(); var targetPos = $("#animatetarget").position(); // Event handler on the button to animate the image // from source location to target location $(buttonHandle).click(function () { $('#sun').animate({ left: targetPos.left - sourcePos.left, top: targetPos.top - sourcePos.top }, 1250, function () { }); }); </script> </body> </html>