This sample shows how to setup a basic JQuery popdown menu embedded in an asp.net webforms application, and how to position it correctly under your menu bar. As you will discover, no code behind at all is needed to make the basic functionality work, so no need for postbacks and displaying and hiding panels. The framework for displaying and hiding the menu is 100% html, css and jquery. The actual content in to show in the menu’s can be set up in any way you want, either with normal links or for example with a datalist or datarepeater that is fed from the code behind.
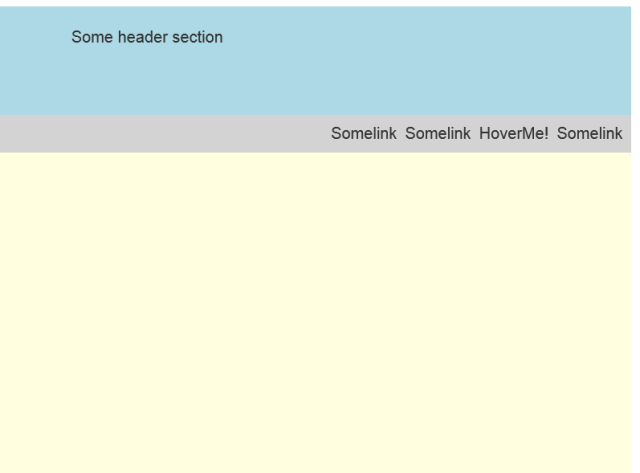
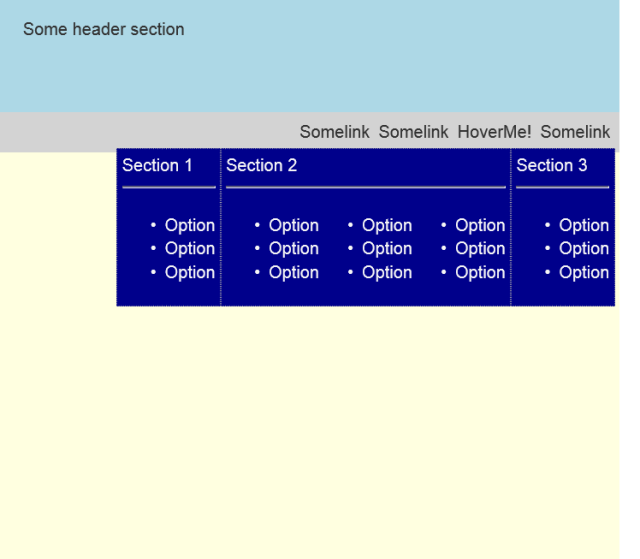
The left image shows out menu bar with the menu hidden. The right image shows the menu displayed over other content when the mouse is moved over the relevant link. The menu block is right aligned within the style of the containing website. The basic flow for the containing site is made with a few tags to setup the columns from WEBFW. You dan download it here if you want to use it. Additionaly, the folowing extra tags for out popup menu are defined in a seperate css:
.menuoption1Link { margin: 4px; } .menuoption2Link { margin: 4px; } .menuoption3Link { margin: 4px; } .menuoption4Link { margin: 4px; } .panelBlockMenu { z-index: 1; position: relative; } .menuSection { padding: 4px; float: left; border: 1px dotted darkgray; background: darkblue; color: whitesmoke; } .optionList { float: left; }
The important tags are panelBlockMenu with the z-index set to make it hover over the content, and the following code:
<!-- Scripting --> <script type="text/javascript"> $(".menuoption3Link").hover(function() { $(".panelBlockMenu").show(); }); $(".panelBlockMenu").mouseleave(function() { $(".panelBlockMenu").hide(); }); </script> <!-- End scripting -->
The first $ tag hooks into the menuoption3Link to make the actual panelBlockMenu visible. The second $ tags hooks into the menu itsself to hide it once the mouse leaves the area. The full markup is as followes:
<%@ Page Language="C#" AutoEventWireup="true" CodeBehind="Default.aspx.cs" Inherits="MenuBarProtoType.Default" %> <!DOCTYPE html> <html xmlns="http://www.w3.org/1999/xhtml"> <head runat="server"> <title></title> <link href="d/css/WebFW041.css" rel="stylesheet" type="text/css" /> <link href="d/css/MenuStyle.css" rel="stylesheet" type="text/css" /> </head> <body> <form id="form1" runat="server"> <!-- Install nuget packages jquery and jquesry scriptmanager to have jqeury at the ready line --> <asp:ScriptManager runat="server"> <Scripts> <asp:ScriptReference Name="jquery" /> </Scripts> </asp:ScriptManager> <div class="width2-12"> </div> <div class="width8-12"> <div class="blockContent" style="background-color: lightblue; height: 100px;"> <div class="alignCenter"><p></p>Some header section</div> </div> <!-- menu bar wrapper --> <div class="blockContent" style="background-color: lightgray"> <!-- menu block --> <div class="alignRight"> <div class="menuoption1Link alignLeft">Somelink</div> <div class="menuoption2Link alignLeft">Somelink</div> <div class="menuoption3Link alignLeft">HoverMe!</div> <div class="menuoption4Link alignLeft">Somelink</div> </div> <div class="alignClear"></div> <div class="alignRight"> <div class="panelBlockMenu" style="display: none;"> <div class="menuSection"> Section 1<hr/> <div class="optionList"> <ul> <li>Option</li> <li>Option</li> <li>Option</li> </ul> </div> </div> <div class="menuSection"> Section 2<hr/> <div class="optionList"> <ul> <li>Option</li> <li>Option</li> <li>Option</li> </ul> </div> <div class="optionList"> <ul> <li>Option</li> <li>Option</li> <li>Option</li> </ul> </div> <div class="optionList"> <ul> <li>Option</li> <li>Option</li> <li>Option</li> </ul> </div> </div> <div class="menuSection"> Section 3<hr/> <div class="optionList"> <ul> <li>Option</li> <li>Option</li> <li>Option</li> </ul> </div> </div> </div> </div> </div> <div class="blockContent" style="background-color: lightyellow; height: 600px;"></div> </div> <div class="width2-12"> </div> <!-- Scripting --> <script type="text/javascript"> $(".menuoption3Link").hover(function() { $(".panelBlockMenu").show(); }); $(".panelBlockMenu").mouseleave(function() { $(".panelBlockMenu").hide(); }); </script> <!-- End scripting --> </form> </body> </html>